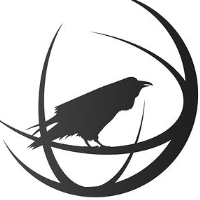
Wednesday, April 23, 2014 6:07:44 PM
poly code
===========================
Y worry Murray Member Level Wednesday, 04/23/14 02:33:59 PM
Re: spdpro post# 2715
Post # of 2725
Not hidden as far as I can see:
=============================
#region Using declarations
using System;
using System.ComponentModel;
using System.Diagnostics;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Xml.Serialization;
using NinjaTrader.Cbi;
using NinjaTrader.Data;
using NinjaTrader.Gui.Chart;
#endregion
// This namespace holds all indicators and is required. Do not change it.
namespace NinjaTrader.Indicator
{
/// <summary>
/// Polnomial Regression Channel
/// </summary>
[Description("Polynomial Regression Channel")]
//port of indicator from MQL4 to NinjaTrader. Original author: fxcanada
// http://codebase.mql4.com/4332
[Gui.Design.DisplayName("PRC2")]
public class PRC2 : Indicator
{
#region Variables
// Wizard generated variables
private int degree = 3; // Default setting for Degree
private int period = 105; // Default setting for Period
private double strdDev = 1.62; // Default setting for StrdDev
private double strdDev2 = 2.000; // Default setting for StrdDev
// User defined variables (add any user defined variables below)
private double[,] ai = new double[10,10];
private double[] b = new double[10];
private double[] x = new double[10];
private double[] sx = new double[10];
private double sum;
private int ip;
private int p;
private int n;
private int f;
private double qq;
private double mm;
private double tt;
private int ii;
private int jj;
private int kk;
private int ll;
private int nn;
private double sq;
private double sq2;
private int i0 = 0;
private int mi;
private int soundsObOs = 0;
private int soundsTEMAS = 0;
private bool Standard = true;
private bool fillZones = false;
private bool showLine = false;
private bool showEMAs = false;
private int zLHATEMAperiod = 34;
private int zLTEMAperiod = 34;
private Color fillColor = Color.Yellow;
#endregion
/// <summary>
/// This method is used to configure the indicator and is called once before any bar data is loaded.
/// </summary>
protected override void Initialize()
{
Add(new Plot(new Pen(Color.DimGray, 2), PlotStyle.Line, "Fx"));//Plot0
Add(new Plot(Color.FromKnownColor(KnownColor.Red), PlotStyle.Line, "Sqh"));//Plot1
Add(new Plot(Color.FromKnownColor(KnownColor.Blue), PlotStyle.Line, "Sql"));//Plot2
Add(new Plot(Color.FromKnownColor(KnownColor.Red), PlotStyle.Line, "Sqh2"));//Plot3
Add(new Plot(Color.FromKnownColor(KnownColor.Blue), PlotStyle.Line, "Sql2"));//Plot4
Plots[0].Pen.DashStyle = DashStyle.DashDot;
Add(new Plot(new Pen(Color.White, 2), PlotStyle.Line, "TEMAplot"));//Plot5
Add(new Plot(new Pen(Color.Black, 2), PlotStyle.Line, "HATEMAplot"));//Plot6
CalculateOnBarClose = false;
Overlay = true;
PriceTypeSupported = false;
PaintPriceMarkers = false;
}
/// <summary>
/// Called on each bar update event (incoming tick)
/// </summary>
protected override void OnBarUpdate()
{
if( CurrentBar < period)return;
ip = period;
p = ip;
sx[1] = p + 1;
nn = degree + 1;
//----------------------sx-------------------------------------------------------------------
for(mi=1;mi<=nn*2-2;mi++) //
{
sum=0;
for(n=i0;n<=i0+p;n++)
{
sum+=Math.Pow(n,mi);
}
sx[mi+1]=sum;
}
//----------------------syx-----------
for(mi=1;mi<=nn;mi++)
{
sum=0.00000;
for(n=i0;n<=i0+p;n++)
{
if(mi==1) sum+=Close[n];
else sum+=Close[n]*Math.Pow(n,mi-1);
}
b[mi]=sum;
}
//===============Matrix=======================================================================================================
for(jj=1;jj<=nn;jj++)
{
for(ii=1; ii<=nn; ii++)
{
kk=ii+jj-1;
ai[ii,jj]=sx[kk];
}
}
//===============Gauss========================================================================================================
for(kk=1; kk<=nn-1; kk++)
{
ll=0;
mm=0;
for(ii=kk; ii<=nn; ii++)
{
if(Math.Abs(ai[ii,kk])>mm)
{
mm=Math.Abs(ai[ii,kk]);
ll=ii;
}
}
if(ll==0) return;
if (ll!=kk)
{
for(jj=1; jj<=nn; jj++)
{
tt=ai[kk,jj];
ai[kk,jj]=ai[ll,jj];
ai[ll,jj]=tt;
}
tt=b[kk];
b[kk]=b[ll];
b[ll]=tt;
}
for(ii=kk+1;ii<=nn;ii++)
{
qq=ai[ii,kk]/ai[kk,kk];
for(jj=1;jj<=nn;jj++)
{
if(jj==kk) ai[ii,jj]=0;
else ai[ii,jj]=ai[ii,jj]-qq*ai[kk,jj];
}
b[ii]=b[ii]-qq*b[kk];
}
}
x[nn]=b[nn]/ai[nn,nn];
for(ii=nn-1;ii>=1;ii--)
{
tt=0;
for(jj=1;jj<=nn-ii;jj++)
{
tt=tt+ai[ii,ii+jj]*x[ii+jj];
x[ii]=(1/ai[ii,ii])*(b[ii]-tt);
}
}
//===========================================================================================================================
for(n=i0;n<=i0+p;n++)
{
sum=0;
for(kk=1;kk<=degree;kk++)
{
sum+=x[kk+1]*Math.Pow(n,kk);
}
Fx.Set(n,x[1]+sum);
}
//-----------------------------------Std-----------------------------------------------------------------------------------
sq=0.0;
for(n=i0;n<=i0+p;n++)
{
sq+=Math.Pow(Close[n]-Fx[n],2);
}
sq=Math.Sqrt(sq/(p+1))*strdDev;
for(n=i0;n<=i0+p;n++)
{
Sqh.Set(n,Fx[n]+sq);
Sql.Set(n,Fx[n]-sq);
}
if(showLine)
{
sq2=0.0;
for(n=i0;n<=i0+p;n++)
{
sq2+=Math.Pow(Close[n]-Fx[n],2);
}
sq2=Math.Sqrt(sq2/(p+1))*strdDev2;
for(n=i0;n<=i0+p;n++)
{
Sqh2.Set(n,Fx[n]+sq2);
Sql2.Set(n,Fx[n]-sq2);
}
}
//----------EMA's
if(showEMAs)
{Values[5].Set(ZeroLagTEMAandHATEMA(zLTEMAperiod,zLHATEMAperiod).ZeroTEMA[0]);//TEMA plot
Values[6].Set(ZeroLagTEMAandHATEMA(zLTEMAperiod,zLHATEMAperiod).ZeroHATEMA[0]);}//HATEMA plot
//----------Fill Zones
if(fillZones && showLine)
{
DrawRegion("HiZone", CurrentBar, 0, Sqh, Sqh2, Color.Empty, fillColor, 2);
DrawRegion("LoZone", CurrentBar, 0, Sql, Sql2, Color.Empty, fillColor, 2);
}
//----------Alerts ObOs or TEMAs cross
if(CrossAbove(Close, Sqh, 1) && soundsObOs > 0 || CrossBelow(Close, Sql, 1) && soundsObOs > 0 || CrossAbove(TEMAplot, HATEMAplot, 1) && soundsTEMAS > 0 || CrossBelow(TEMAplot, HATEMAplot, 1) && soundsTEMAS > 0)
{if(Standard)
{if(soundsObOs == 1)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\chimes.wav",4,fillColor,Color.Black);}
if(soundsTEMAS == 1)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\chimes.wav",4,Color.Black,Color.White);}
if(soundsObOs == 2)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\ding.wav",4,fillColor,Color.Black);}
if(soundsTEMAS == 2)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\ding.wav",4,Color.Black,Color.White);}
if(soundsObOs == 3)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","alert4.wav",4,fillColor,Color.Black);}
if(soundsTEMAS == 3)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","alert4.wav",4,Color.Black,Color.White);}}
else
{if(soundsObOs == 1)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\chimes.wav",15,fillColor,Color.Black);
PlaySound(@"c:\\windows\\media\\chimes.wav");}
if(soundsTEMAS == 1)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\chimes.wav",15,Color.Black,Color.White);
PlaySound(@"c:\\windows\\media\\chimes.wav");}
if(soundsObOs == 2)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\ding.wav",15,fillColor,Color.Black);
PlaySound(@"c:\\windows\\media\\ding.wav");}
if(soundsTEMAS == 2)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\ding.wav",15,Color.Black,Color.White);
PlaySound(@"c:\\windows\\media\\ding.wav");}
if(soundsObOs == 3)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","alert4.wav",15,fillColor,Color.Black);
PlaySound(@"\\alert4.wav");}
if(soundsTEMAS == 3)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","alert4.wav",15,Color.Black,Color.White);
PlaySound(@"\\alert4.wav");}}
}
}
#region Properties
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Fx
{
get { return Values[0]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Sqh
{
get { return Values[1]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Sql
{
get { return Values[2]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Sqh2
{
get { return Values[3]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Sql2
{
get { return Values[4]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries TEMAplot
{
get { return Values[5]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries HATEMAplot
{
get { return Values[6]; }
}
[Description("type of polynomial 1, 2, 3 or 4")]
[Category("Parameters")]
public int Degree
{
get { return degree; }
set { degree = Math.Min(4,Math.Max(value,1)); }
}
[Description("nmbr of bars to use in calculation")]
[Category("Parameters")]
public int Period
{
get { return period; }
set { period = Math.Max(1, value); }
}
[Description("Standard Deviations")]
[Category("Parameters")]
public double StrdDev
{
get { return strdDev; }
set { strdDev = Math.Max(0.5, value); }
}
[Description("Standard Deviations")]
[Category("Parameters")]
public double StrdDev2
{
get { return strdDev2; }
set { strdDev2 = Math.Max(0.5, value); }
}
[Description("ZeroLagHATEMA Period")]
[Category("Parameters")]
public int ZLHATEMAperiod
{
get { return zLHATEMAperiod; }
set { zLHATEMAperiod = Math.Max(1, value); }
}
[Description("ZeroLagTEMA Period")]
[Category("Parameters")]
public int ZLTEMAperiod
{
get { return zLTEMAperiod; }
set { zLTEMAperiod = Math.Max(1, value); }
}
[Description("Sound selection for cross into ObOs areas: 0 = no sound, 1 = Chimes, 2 = Ding, 3 = Alert4(NT)")]
[Gui.Design.DisplayName ("\t\tSound ObOs X-ing")]
[Category("Options")]
public int SoundsObOs
{
get { return soundsObOs; }
set { soundsObOs = Math.Min(3,Math.Max(value,0)); }
}
[Description("Sound selection for cross of EMA's: 0 = no sound, 1 = Chimes, 2 = Ding, 3 = Alert4(NT)")]
[Gui.Design.DisplayName ("\t\tSound EMA X-ing")]
[Category("Options")]
public int SoundsTEMAS
{
get { return soundsTEMAS; }
set { soundsTEMAS = Math.Min(3,Math.Max(value,0)); }
}
[Description("Standard alert (true) or constant sound barrage (false)")]
[Gui.Design.DisplayName ("\t\tStandard Alert?")]
[Category("Options")]
public bool standard
{
get { return Standard; }
set { Standard = value; }
}
[Description("Fill the zones created by the two Sqh and Sql lines")]
[Gui.Design.DisplayName ("Fill OB/OS zones?")]
[Category("Options")]
public bool FillZones
{
get { return fillZones; }
set { fillZones = value; }
}
[Description("This will plot the second set of lines which then gives the option of filling the zone")]
[Gui.Design.DisplayName ("\tPlot Sqh2 and Sql2 lines?")]
[Category("Options")]
public bool ShowLine
{
get { return showLine; }
set { showLine = value; }
}
[Description("This will plot ZeroLagTEMA and ZeroLagHATEMA moving averages")]
[Gui.Design.DisplayName ("\tPlot ZLTEMA and ZLHATEMA?")]
[Category("Options")]
public bool ShowEMAs
{
get { return showEMAs; }
set { showEMAs = value; }
}
[Browsable(false)]
public string fillColorSerialize
{
get { return NinjaTrader.Gui.Design.SerializableColor.ToString(fillColor); }
set { fillColor = NinjaTrader.Gui.Design.SerializableColor.FromString(value); }
}
[XmlIgnore()]
[Description("Fill zone color.")]
[Category("Options")]
[Gui.Design.DisplayNameAttribute("Fill zone color")]
public Color FillColor
{
get { return fillColor; }
set { fillColor = value; }
}
#endregion
}
}
#region NinjaScript generated code. Neither change nor remove.
// This namespace holds all indicators and is required. Do not change it.
namespace NinjaTrader.Indicator
{
public partial class Indicator : IndicatorBase
{
private PRC2[] cachePRC2 = null;
private static PRC2 checkPRC2 = new PRC2();
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
public PRC2 PRC2(int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
return PRC2(Input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
public PRC2 PRC2(Data.IDataSeries input, int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
if (cachePRC2 != null)
for (int idx = 0; idx < cachePRC2.Length; idx++)
if (cachePRC2[idx].Degree == degree && cachePRC2[idx].Period == period && Math.Abs(cachePRC2[idx].StrdDev - strdDev) <= double.Epsilon && Math.Abs(cachePRC2[idx].StrdDev2 - strdDev2) <= double.Epsilon && cachePRC2[idx].ZLHATEMAperiod == zLHATEMAperiod && cachePRC2[idx].ZLTEMAperiod == zLTEMAperiod && cachePRC2[idx].EqualsInput(input))
return cachePRC2[idx];
lock (checkPRC2)
{
checkPRC2.Degree = degree;
degree = checkPRC2.Degree;
checkPRC2.Period = period;
period = checkPRC2.Period;
checkPRC2.StrdDev = strdDev;
strdDev = checkPRC2.StrdDev;
checkPRC2.StrdDev2 = strdDev2;
strdDev2 = checkPRC2.StrdDev2;
checkPRC2.ZLHATEMAperiod = zLHATEMAperiod;
zLHATEMAperiod = checkPRC2.ZLHATEMAperiod;
checkPRC2.ZLTEMAperiod = zLTEMAperiod;
zLTEMAperiod = checkPRC2.ZLTEMAperiod;
if (cachePRC2 != null)
for (int idx = 0; idx < cachePRC2.Length; idx++)
if (cachePRC2[idx].Degree == degree && cachePRC2[idx].Period == period && Math.Abs(cachePRC2[idx].StrdDev - strdDev) <= double.Epsilon && Math.Abs(cachePRC2[idx].StrdDev2 - strdDev2) <= double.Epsilon && cachePRC2[idx].ZLHATEMAperiod == zLHATEMAperiod && cachePRC2[idx].ZLTEMAperiod == zLTEMAperiod && cachePRC2[idx].EqualsInput(input))
return cachePRC2[idx];
PRC2 indicator = new PRC2();
indicator.BarsRequired = BarsRequired;
indicator.CalculateOnBarClose = CalculateOnBarClose;
#if NT7
indicator.ForceMaximumBarsLookBack256 = ForceMaximumBarsLookBack256;
indicator.MaximumBarsLookBack = MaximumBarsLookBack;
#endif
indicator.Input = input;
indicator.Degree = degree;
indicator.Period = period;
indicator.StrdDev = strdDev;
indicator.StrdDev2 = strdDev2;
indicator.ZLHATEMAperiod = zLHATEMAperiod;
indicator.ZLTEMAperiod = zLTEMAperiod;
Indicators.Add(indicator);
indicator.SetUp();
PRC2[] tmp = new PRC2[cachePRC2 == null ? 1 : cachePRC2.Length + 1];
if (cachePRC2 != null)
cachePRC2.CopyTo(tmp, 0);
tmp[tmp.Length - 1] = indicator;
cachePRC2 = tmp;
return indicator;
}
}
}
}
// This namespace holds all market analyzer column definitions and is required. Do not change it.
namespace NinjaTrader.MarketAnalyzer
{
public partial class Column : ColumnBase
{
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
[Gui.Design.WizardCondition("Indicator")]
public Indicator.PRC2 PRC2(int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
return _indicator.PRC2(Input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
public Indicator.PRC2 PRC2(Data.IDataSeries input, int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
return _indicator.PRC2(input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
}
}
// This namespace holds all strategies and is required. Do not change it.
namespace NinjaTrader.Strategy
{
public partial class Strategy : StrategyBase
{
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
[Gui.Design.WizardCondition("Indicator")]
public Indicator.PRC2 PRC2(int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
return _indicator.PRC2(Input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
public Indicator.PRC2 PRC2(Data.IDataSeries input, int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
if (InInitialize && input == null)
throw new ArgumentException("You only can access an indicator with the default input/bar series from within the 'Initialize()' method");
return _indicator.PRC2(input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
}
}
#endregion
===========================
Y worry Murray Member Level Wednesday, 04/23/14 02:33:59 PM
Re: spdpro post# 2715
Post # of 2725
Not hidden as far as I can see:
=============================
#region Using declarations
using System;
using System.ComponentModel;
using System.Diagnostics;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Xml.Serialization;
using NinjaTrader.Cbi;
using NinjaTrader.Data;
using NinjaTrader.Gui.Chart;
#endregion
// This namespace holds all indicators and is required. Do not change it.
namespace NinjaTrader.Indicator
{
/// <summary>
/// Polnomial Regression Channel
/// </summary>
[Description("Polynomial Regression Channel")]
//port of indicator from MQL4 to NinjaTrader. Original author: fxcanada
// http://codebase.mql4.com/4332
[Gui.Design.DisplayName("PRC2")]
public class PRC2 : Indicator
{
#region Variables
// Wizard generated variables
private int degree = 3; // Default setting for Degree
private int period = 105; // Default setting for Period
private double strdDev = 1.62; // Default setting for StrdDev
private double strdDev2 = 2.000; // Default setting for StrdDev
// User defined variables (add any user defined variables below)
private double[,] ai = new double[10,10];
private double[] b = new double[10];
private double[] x = new double[10];
private double[] sx = new double[10];
private double sum;
private int ip;
private int p;
private int n;
private int f;
private double qq;
private double mm;
private double tt;
private int ii;
private int jj;
private int kk;
private int ll;
private int nn;
private double sq;
private double sq2;
private int i0 = 0;
private int mi;
private int soundsObOs = 0;
private int soundsTEMAS = 0;
private bool Standard = true;
private bool fillZones = false;
private bool showLine = false;
private bool showEMAs = false;
private int zLHATEMAperiod = 34;
private int zLTEMAperiod = 34;
private Color fillColor = Color.Yellow;
#endregion
/// <summary>
/// This method is used to configure the indicator and is called once before any bar data is loaded.
/// </summary>
protected override void Initialize()
{
Add(new Plot(new Pen(Color.DimGray, 2), PlotStyle.Line, "Fx"));//Plot0
Add(new Plot(Color.FromKnownColor(KnownColor.Red), PlotStyle.Line, "Sqh"));//Plot1
Add(new Plot(Color.FromKnownColor(KnownColor.Blue), PlotStyle.Line, "Sql"));//Plot2
Add(new Plot(Color.FromKnownColor(KnownColor.Red), PlotStyle.Line, "Sqh2"));//Plot3
Add(new Plot(Color.FromKnownColor(KnownColor.Blue), PlotStyle.Line, "Sql2"));//Plot4
Plots[0].Pen.DashStyle = DashStyle.DashDot;
Add(new Plot(new Pen(Color.White, 2), PlotStyle.Line, "TEMAplot"));//Plot5
Add(new Plot(new Pen(Color.Black, 2), PlotStyle.Line, "HATEMAplot"));//Plot6
CalculateOnBarClose = false;
Overlay = true;
PriceTypeSupported = false;
PaintPriceMarkers = false;
}
/// <summary>
/// Called on each bar update event (incoming tick)
/// </summary>
protected override void OnBarUpdate()
{
if( CurrentBar < period)return;
ip = period;
p = ip;
sx[1] = p + 1;
nn = degree + 1;
//----------------------sx-------------------------------------------------------------------
for(mi=1;mi<=nn*2-2;mi++) //
{
sum=0;
for(n=i0;n<=i0+p;n++)
{
sum+=Math.Pow(n,mi);
}
sx[mi+1]=sum;
}
//----------------------syx-----------
for(mi=1;mi<=nn;mi++)
{
sum=0.00000;
for(n=i0;n<=i0+p;n++)
{
if(mi==1) sum+=Close[n];
else sum+=Close[n]*Math.Pow(n,mi-1);
}
b[mi]=sum;
}
//===============Matrix=======================================================================================================
for(jj=1;jj<=nn;jj++)
{
for(ii=1; ii<=nn; ii++)
{
kk=ii+jj-1;
ai[ii,jj]=sx[kk];
}
}
//===============Gauss========================================================================================================
for(kk=1; kk<=nn-1; kk++)
{
ll=0;
mm=0;
for(ii=kk; ii<=nn; ii++)
{
if(Math.Abs(ai[ii,kk])>mm)
{
mm=Math.Abs(ai[ii,kk]);
ll=ii;
}
}
if(ll==0) return;
if (ll!=kk)
{
for(jj=1; jj<=nn; jj++)
{
tt=ai[kk,jj];
ai[kk,jj]=ai[ll,jj];
ai[ll,jj]=tt;
}
tt=b[kk];
b[kk]=b[ll];
b[ll]=tt;
}
for(ii=kk+1;ii<=nn;ii++)
{
qq=ai[ii,kk]/ai[kk,kk];
for(jj=1;jj<=nn;jj++)
{
if(jj==kk) ai[ii,jj]=0;
else ai[ii,jj]=ai[ii,jj]-qq*ai[kk,jj];
}
b[ii]=b[ii]-qq*b[kk];
}
}
x[nn]=b[nn]/ai[nn,nn];
for(ii=nn-1;ii>=1;ii--)
{
tt=0;
for(jj=1;jj<=nn-ii;jj++)
{
tt=tt+ai[ii,ii+jj]*x[ii+jj];
x[ii]=(1/ai[ii,ii])*(b[ii]-tt);
}
}
//===========================================================================================================================
for(n=i0;n<=i0+p;n++)
{
sum=0;
for(kk=1;kk<=degree;kk++)
{
sum+=x[kk+1]*Math.Pow(n,kk);
}
Fx.Set(n,x[1]+sum);
}
//-----------------------------------Std-----------------------------------------------------------------------------------
sq=0.0;
for(n=i0;n<=i0+p;n++)
{
sq+=Math.Pow(Close[n]-Fx[n],2);
}
sq=Math.Sqrt(sq/(p+1))*strdDev;
for(n=i0;n<=i0+p;n++)
{
Sqh.Set(n,Fx[n]+sq);
Sql.Set(n,Fx[n]-sq);
}
if(showLine)
{
sq2=0.0;
for(n=i0;n<=i0+p;n++)
{
sq2+=Math.Pow(Close[n]-Fx[n],2);
}
sq2=Math.Sqrt(sq2/(p+1))*strdDev2;
for(n=i0;n<=i0+p;n++)
{
Sqh2.Set(n,Fx[n]+sq2);
Sql2.Set(n,Fx[n]-sq2);
}
}
//----------EMA's
if(showEMAs)
{Values[5].Set(ZeroLagTEMAandHATEMA(zLTEMAperiod,zLHATEMAperiod).ZeroTEMA[0]);//TEMA plot
Values[6].Set(ZeroLagTEMAandHATEMA(zLTEMAperiod,zLHATEMAperiod).ZeroHATEMA[0]);}//HATEMA plot
//----------Fill Zones
if(fillZones && showLine)
{
DrawRegion("HiZone", CurrentBar, 0, Sqh, Sqh2, Color.Empty, fillColor, 2);
DrawRegion("LoZone", CurrentBar, 0, Sql, Sql2, Color.Empty, fillColor, 2);
}
//----------Alerts ObOs or TEMAs cross
if(CrossAbove(Close, Sqh, 1) && soundsObOs > 0 || CrossBelow(Close, Sql, 1) && soundsObOs > 0 || CrossAbove(TEMAplot, HATEMAplot, 1) && soundsTEMAS > 0 || CrossBelow(TEMAplot, HATEMAplot, 1) && soundsTEMAS > 0)
{if(Standard)
{if(soundsObOs == 1)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\chimes.wav",4,fillColor,Color.Black);}
if(soundsTEMAS == 1)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\chimes.wav",4,Color.Black,Color.White);}
if(soundsObOs == 2)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\ding.wav",4,fillColor,Color.Black);}
if(soundsTEMAS == 2)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\ding.wav",4,Color.Black,Color.White);}
if(soundsObOs == 3)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","alert4.wav",4,fillColor,Color.Black);}
if(soundsTEMAS == 3)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","alert4.wav",4,Color.Black,Color.White);}}
else
{if(soundsObOs == 1)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\chimes.wav",15,fillColor,Color.Black);
PlaySound(@"c:\\windows\\media\\chimes.wav");}
if(soundsTEMAS == 1)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\chimes.wav",15,Color.Black,Color.White);
PlaySound(@"c:\\windows\\media\\chimes.wav");}
if(soundsObOs == 2)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\ding.wav",15,fillColor,Color.Black);
PlaySound(@"c:\\windows\\media\\ding.wav");}
if(soundsTEMAS == 2)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","c:\\windows\\media\\ding.wav",15,Color.Black,Color.White);
PlaySound(@"c:\\windows\\media\\ding.wav");}
if(soundsObOs == 3)
{Alert("Price/ObOs Cross", NinjaTrader.Cbi.Priority.High,"Price/ObOs Cross " + Bars.Period.ToString() + " chart","alert4.wav",15,fillColor,Color.Black);
PlaySound(@"\\alert4.wav");}
if(soundsTEMAS == 3)
{Alert("TEMA Cross", NinjaTrader.Cbi.Priority.High,"TEMA Cross " + Bars.Period.ToString() + " chart","alert4.wav",15,Color.Black,Color.White);
PlaySound(@"\\alert4.wav");}}
}
}
#region Properties
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Fx
{
get { return Values[0]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Sqh
{
get { return Values[1]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Sql
{
get { return Values[2]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Sqh2
{
get { return Values[3]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries Sql2
{
get { return Values[4]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries TEMAplot
{
get { return Values[5]; }
}
[Browsable(false)] // this line prevents the data series from being displayed in the indicator properties dialog, do not remove
[XmlIgnore()] // this line ensures that the indicator can be saved/recovered as part of a chart template, do not remove
public DataSeries HATEMAplot
{
get { return Values[6]; }
}
[Description("type of polynomial 1, 2, 3 or 4")]
[Category("Parameters")]
public int Degree
{
get { return degree; }
set { degree = Math.Min(4,Math.Max(value,1)); }
}
[Description("nmbr of bars to use in calculation")]
[Category("Parameters")]
public int Period
{
get { return period; }
set { period = Math.Max(1, value); }
}
[Description("Standard Deviations")]
[Category("Parameters")]
public double StrdDev
{
get { return strdDev; }
set { strdDev = Math.Max(0.5, value); }
}
[Description("Standard Deviations")]
[Category("Parameters")]
public double StrdDev2
{
get { return strdDev2; }
set { strdDev2 = Math.Max(0.5, value); }
}
[Description("ZeroLagHATEMA Period")]
[Category("Parameters")]
public int ZLHATEMAperiod
{
get { return zLHATEMAperiod; }
set { zLHATEMAperiod = Math.Max(1, value); }
}
[Description("ZeroLagTEMA Period")]
[Category("Parameters")]
public int ZLTEMAperiod
{
get { return zLTEMAperiod; }
set { zLTEMAperiod = Math.Max(1, value); }
}
[Description("Sound selection for cross into ObOs areas: 0 = no sound, 1 = Chimes, 2 = Ding, 3 = Alert4(NT)")]
[Gui.Design.DisplayName ("\t\tSound ObOs X-ing")]
[Category("Options")]
public int SoundsObOs
{
get { return soundsObOs; }
set { soundsObOs = Math.Min(3,Math.Max(value,0)); }
}
[Description("Sound selection for cross of EMA's: 0 = no sound, 1 = Chimes, 2 = Ding, 3 = Alert4(NT)")]
[Gui.Design.DisplayName ("\t\tSound EMA X-ing")]
[Category("Options")]
public int SoundsTEMAS
{
get { return soundsTEMAS; }
set { soundsTEMAS = Math.Min(3,Math.Max(value,0)); }
}
[Description("Standard alert (true) or constant sound barrage (false)")]
[Gui.Design.DisplayName ("\t\tStandard Alert?")]
[Category("Options")]
public bool standard
{
get { return Standard; }
set { Standard = value; }
}
[Description("Fill the zones created by the two Sqh and Sql lines")]
[Gui.Design.DisplayName ("Fill OB/OS zones?")]
[Category("Options")]
public bool FillZones
{
get { return fillZones; }
set { fillZones = value; }
}
[Description("This will plot the second set of lines which then gives the option of filling the zone")]
[Gui.Design.DisplayName ("\tPlot Sqh2 and Sql2 lines?")]
[Category("Options")]
public bool ShowLine
{
get { return showLine; }
set { showLine = value; }
}
[Description("This will plot ZeroLagTEMA and ZeroLagHATEMA moving averages")]
[Gui.Design.DisplayName ("\tPlot ZLTEMA and ZLHATEMA?")]
[Category("Options")]
public bool ShowEMAs
{
get { return showEMAs; }
set { showEMAs = value; }
}
[Browsable(false)]
public string fillColorSerialize
{
get { return NinjaTrader.Gui.Design.SerializableColor.ToString(fillColor); }
set { fillColor = NinjaTrader.Gui.Design.SerializableColor.FromString(value); }
}
[XmlIgnore()]
[Description("Fill zone color.")]
[Category("Options")]
[Gui.Design.DisplayNameAttribute("Fill zone color")]
public Color FillColor
{
get { return fillColor; }
set { fillColor = value; }
}
#endregion
}
}
#region NinjaScript generated code. Neither change nor remove.
// This namespace holds all indicators and is required. Do not change it.
namespace NinjaTrader.Indicator
{
public partial class Indicator : IndicatorBase
{
private PRC2[] cachePRC2 = null;
private static PRC2 checkPRC2 = new PRC2();
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
public PRC2 PRC2(int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
return PRC2(Input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
public PRC2 PRC2(Data.IDataSeries input, int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
if (cachePRC2 != null)
for (int idx = 0; idx < cachePRC2.Length; idx++)
if (cachePRC2[idx].Degree == degree && cachePRC2[idx].Period == period && Math.Abs(cachePRC2[idx].StrdDev - strdDev) <= double.Epsilon && Math.Abs(cachePRC2[idx].StrdDev2 - strdDev2) <= double.Epsilon && cachePRC2[idx].ZLHATEMAperiod == zLHATEMAperiod && cachePRC2[idx].ZLTEMAperiod == zLTEMAperiod && cachePRC2[idx].EqualsInput(input))
return cachePRC2[idx];
lock (checkPRC2)
{
checkPRC2.Degree = degree;
degree = checkPRC2.Degree;
checkPRC2.Period = period;
period = checkPRC2.Period;
checkPRC2.StrdDev = strdDev;
strdDev = checkPRC2.StrdDev;
checkPRC2.StrdDev2 = strdDev2;
strdDev2 = checkPRC2.StrdDev2;
checkPRC2.ZLHATEMAperiod = zLHATEMAperiod;
zLHATEMAperiod = checkPRC2.ZLHATEMAperiod;
checkPRC2.ZLTEMAperiod = zLTEMAperiod;
zLTEMAperiod = checkPRC2.ZLTEMAperiod;
if (cachePRC2 != null)
for (int idx = 0; idx < cachePRC2.Length; idx++)
if (cachePRC2[idx].Degree == degree && cachePRC2[idx].Period == period && Math.Abs(cachePRC2[idx].StrdDev - strdDev) <= double.Epsilon && Math.Abs(cachePRC2[idx].StrdDev2 - strdDev2) <= double.Epsilon && cachePRC2[idx].ZLHATEMAperiod == zLHATEMAperiod && cachePRC2[idx].ZLTEMAperiod == zLTEMAperiod && cachePRC2[idx].EqualsInput(input))
return cachePRC2[idx];
PRC2 indicator = new PRC2();
indicator.BarsRequired = BarsRequired;
indicator.CalculateOnBarClose = CalculateOnBarClose;
#if NT7
indicator.ForceMaximumBarsLookBack256 = ForceMaximumBarsLookBack256;
indicator.MaximumBarsLookBack = MaximumBarsLookBack;
#endif
indicator.Input = input;
indicator.Degree = degree;
indicator.Period = period;
indicator.StrdDev = strdDev;
indicator.StrdDev2 = strdDev2;
indicator.ZLHATEMAperiod = zLHATEMAperiod;
indicator.ZLTEMAperiod = zLTEMAperiod;
Indicators.Add(indicator);
indicator.SetUp();
PRC2[] tmp = new PRC2[cachePRC2 == null ? 1 : cachePRC2.Length + 1];
if (cachePRC2 != null)
cachePRC2.CopyTo(tmp, 0);
tmp[tmp.Length - 1] = indicator;
cachePRC2 = tmp;
return indicator;
}
}
}
}
// This namespace holds all market analyzer column definitions and is required. Do not change it.
namespace NinjaTrader.MarketAnalyzer
{
public partial class Column : ColumnBase
{
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
[Gui.Design.WizardCondition("Indicator")]
public Indicator.PRC2 PRC2(int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
return _indicator.PRC2(Input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
public Indicator.PRC2 PRC2(Data.IDataSeries input, int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
return _indicator.PRC2(input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
}
}
// This namespace holds all strategies and is required. Do not change it.
namespace NinjaTrader.Strategy
{
public partial class Strategy : StrategyBase
{
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
[Gui.Design.WizardCondition("Indicator")]
public Indicator.PRC2 PRC2(int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
return _indicator.PRC2(Input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
/// <summary>
/// Polynomial Regression Channel
/// </summary>
/// <returns></returns>
public Indicator.PRC2 PRC2(Data.IDataSeries input, int degree, int period, double strdDev, double strdDev2, int zLHATEMAperiod, int zLTEMAperiod)
{
if (InInitialize && input == null)
throw new ArgumentException("You only can access an indicator with the default input/bar series from within the 'Initialize()' method");
return _indicator.PRC2(input, degree, period, strdDev, strdDev2, zLHATEMAperiod, zLTEMAperiod);
}
}
}
#endregion
Disaster is Only a Keystroke Away!
Join the InvestorsHub Community
Register for free to join our community of investors and share your ideas. You will also get access to streaming quotes, interactive charts, trades, portfolio, live options flow and more tools.